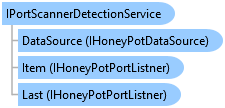
public interface IPortScannerDetectionService : Walter.BOM.IRepositoryStorageLocation
using Microsoft.Extensions.DependencyInjection; using System; public class Example { public static void Main() { // Configure services var serviceProvider = new ServiceCollection() .UsePortScannerProtection(option => { option.Echo = 7; option.IgnoreAfterDetection = IgnoreScope.IPAddressAndPort; }) .BuildServiceProvider(); // Retrieve the port scanner detection service var portScannerService = serviceProvider.GetRequiredService<IPortScannerDetectionService>(); // Subscribe to the OnPortAccessDetected event portScannerService.OnPortAccessDetected += (sender, e) => { Console.WriteLine($"Port access detected: IP={e.IPAddress}, Port={e.Port}"); }; // Loop over each registered listener and add event handlers for detecting port access foreach (var listener in portScannerService.Listeners) { listener.OnDataReceived += OnDataReceived; listener.OnDetecting += OnDetecting; } // Start the service portScannerService.Start(); // Additional logic here } private static void OnDataReceived(object sender, DataReceivedEventArgs e) { Console.WriteLine($"Data received from IP={e.IPAddress}, Port={e.Port}"); } private static void OnDetecting(object sender, DetectingEventArgs e) { Console.WriteLine($"Detecting: IP={e.Detection.IPAddress}, Port={e.Detection.Port}"); } }
Target Platforms: Windows 7, Windows Vista SP1 or later, Windows XP SP3, Windows Server 2008 (Server Core not supported), Windows Server 2008 R2 (Server Core supported with SP1 or later), Windows Server 2003 SP2